Android Basics
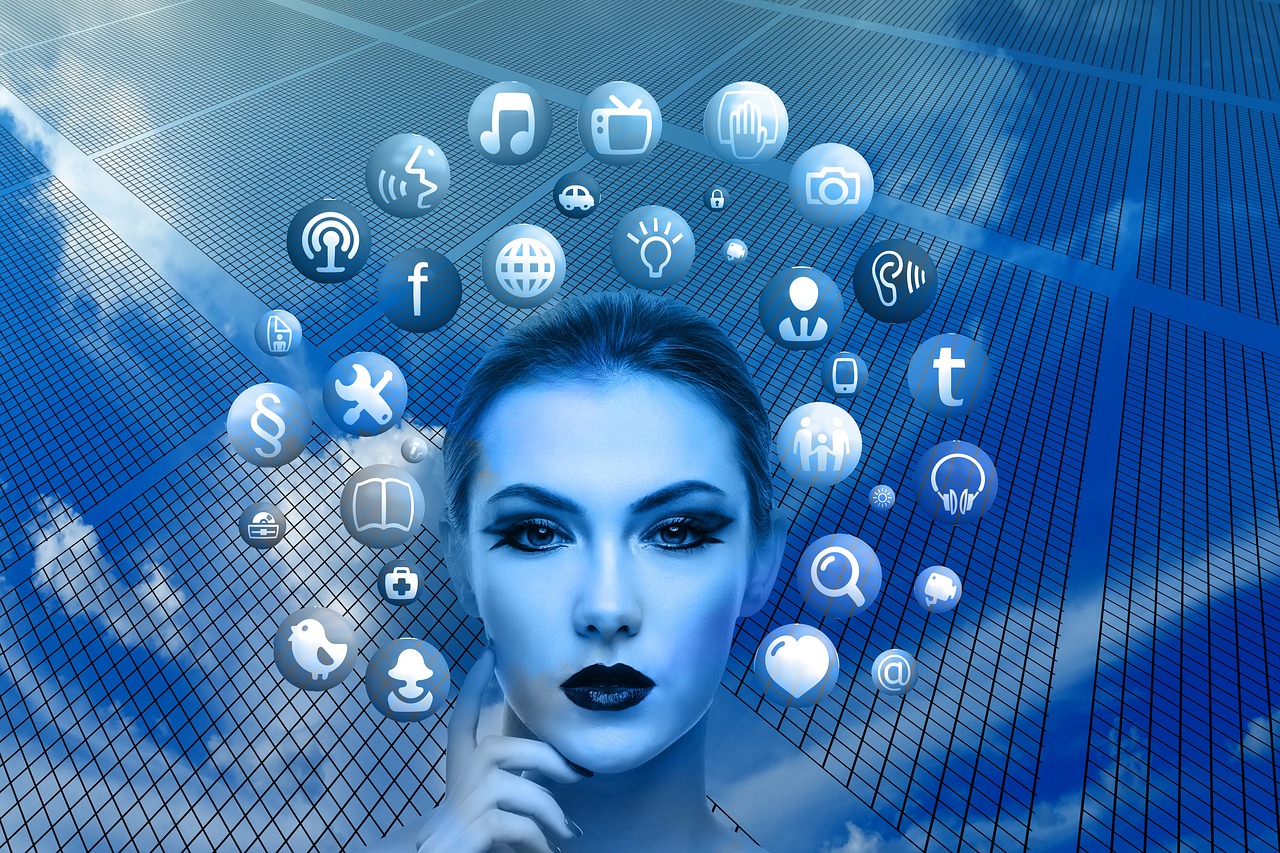
Android is a mobile operating system developed by Google. It is based on the Linux kernel and is designed primarily for touchscreen mobile devices such as smartphones and tablets.
Android is open-source, which means that developers can freely modify and distribute the source code. This has led to a large and active developer community, with a wide range of custom ROMs, kernels, and apps available for Android devices.
public class SortArray { public static void main(String[] args) { int[] arr = {5, 2, 8, 1, 9}; // Selection sort algorithm for (int i = 0; i < arr.length - 1; i++) { int minIndex = i; for (int j = i + 1; j < arr.length; j++) { if (arr[j] < arr[minIndex]) { minIndex = j; } } // Swap elements at minIndex and i int temp = arr[minIndex]; arr[minIndex] = arr[i]; arr[i] = temp; } // Print sorted array for (int num : arr) { System.out.print(num + " "); } } }
In order to develop Android apps, you will need to install the Android SDK (Software Development Kit) and set up an Android development environment on your computer. The Android SDK includes tools and libraries that you can use to build, test, and debug your apps.
There are several tools available for Android development, but the most popular is Android Studio, which is the official integrated development environment (IDE) for Android. It includes a set of tools for building, testing, and debugging Android apps, as well as a graphical user interface (GUI) for designing user interfaces and testing app functionality.
To start learning Android development, you will need to familiarize yourself with the Android framework and learn how to use the Android SDK and Android Studio. There are many online resources available, including official documentation and tutorials, as well as a large number of books and online courses.